Introducing Guise™: The Framework
Guise is more than a toolkit or a library; it is a framework that encompasses your entire application. Guise provides the building blocks for the application to represent itself, its sessions with users, the container into which it is installed, and the platform on which the sessions are running. Guise conceptually supports many types of platforms, including a Windows program, a Java Swing application, or an HTTP client/server arrangement in which user interacts with a web browser. The current implementation of Guise supports the web platform; when other platforms are implemented, your application should run the same on those platforms with little or no modification.
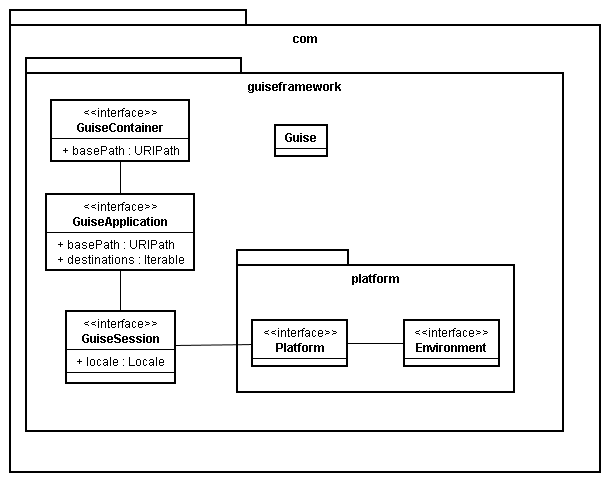
The diagram illustrates the top-level interfaces that comprise the Guise framework domain model. For a JVM instance, there will be a single instance of the Guise
class, providing Guise version and other information. The other interfaces manage your application, user sessions, and interaction with the environment.
Guise Container
The GuiseContainer
interface is the top-most type in the Guise hierarchy. The Guise container manages the the application life cycle, including initialization and uninstallation. The Guise container also provides an absolute URI path, basePath
, that forms the base of all destinations of applications within that container. Most of the time the Guise container does its work automatically, and there is usually no need to interact with it explicitly.
On the web platform, there is one Guise container, an instance of HTTPServletGuiseContainer
, for each JVM web application (i.e. for each instance of ServletContext
.)
Guise Application
Apart from the Guise component hierarchy component hierarchy, the Guise application and session interfaces are the two most important pieces of the Guise domain model. The GuiseApplication
interface encapsulates a single program and manages its destinations and sessions.
Like GuiseContainer
, GuiseApplication
provides a basePath
indicating the base of all application destinations. Like the container base path, the application base path is also absolute (i.e. it begins with the path separator character '/'
); in fact, the application base path begins with the container base path.
Let's assume that on the Guise web platform you deploy your example-application.war
file with the web application mapped to the webapp
path. The example-application.war file contains a web.xml
file, which refers to your application description document example-application.turf
, as illustrated by the following web.xml
excerpt:
<listener>
<description>The class that manages Guise sessions in an HTTP servlet environment.</description>
<listener-class>com.guiseframework.platform.web.HTTPGuiseSessionManager</listener-class>
</listener>
<servlet>
<description>Example Application</description>
<servlet-name>exampleApplication</servlet-name>
<servlet-class>com.guiseframework.platform.web.GuiseHTTPServlet</servlet-class>
<init-param>
<param-name>application</param-name>
<param-value>example-application.turf</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>exampleApplication</servlet-name>
<url-pattern>/example/*</url-pattern>
</servlet-mapping>
This will result in a Guise container base path of /webapp/
and a Guise application base path of /webapp/example/
.
Destinations
A Guise application has a set of Destination
s, each mapped to a particular path relative to the application base path. Each Destination
represents a single point of user interaction in the application. Historically, application presented the user with a single point or "screen" from which all interaction took place. Traditional web applications, at the other extreme, presented a separate "page" for each interaction or even sequence of interactions. Guise provides a the flexibility to provide as many or as few destinations as you need for your application.
The most common destination is the ComponentDestination
, which maps a path to a Component
which will provide interaction with the user at that destination. Destinations are flexible; they can be mapped to regular expressions to match any of several possible paths. Other possible destinations include ResourceReadDestination
, which will return the contents of a resource (or generate contents from scratch), and PermanentRedirectReferenceDestination
, which will redirect permanently to another defined destination.
Application Description Documents
A Guise application is defined within an application description document. This document uses URF PLOOP a framework for decribing resources and their relationships, using the TURF format. The application description document uses simple, consistent data framework, stored in the TURF format. TURF documents begin with the signature
and contain an optional preamble inside between the colon `URF
':'
and semicolon ';'
character; it is here that you will associate namespaces with prefixes, using the tilde character '~'
, to use later in the document. For example, by associating the namespace URI <java:/com/guiseframework/>
with the string "guise"
, you will be able to refer to the class com.guiseframework.DefaultGuiseApplication
using the name guise.DefaultGuiseApplication
instead of writing out the full reference to «java:/com/guiseframework/DefaultGuiseApplication»
.
The body of the TURF document comes between the currency sign '¤'
and the ending full-stop character '.'
. The body of the TURF document contains resource descriptions, and it is here that you will describe your Guise application. In the example below, we'll indicate that the application is an instance of com.guiseframework.DefaultGuiseApplication
by placing a reference to that class after an asterisk: *guise.DefaultGuiseApplication
.
`URF:
"guise"~<java:/com/guiseframework/>,
;¤
*guise.DefaultGuiseApplication
.
Describe the application by placing property-value pairs between the colon ':'
and semicolon ';'
characters. Each property is the name of a property setter. The method GuiseApplication.setDestinations(java.util.List<Destination>)
indicates that there is a property destinations
which has a list as a value. In TURF, a list is indicated by brackets '['
and ']'
, and contain other resource descriptions. Below we provide a list of a single destination, a component destination that maps the path helloWorld
to the component com.example.HelloWorldPanel
. In TURF, the parentheses characers '('
and ')'
indicate constructor arguments for a class; here they indicate arguments for the ComponentDestination(com.garretwilson.net.URIPath path, java.lang.Class<Component> componentClass)
constructor.
`URF:
"guise"~<java:/com/guiseframework/>,
"example"~<java:/com/example/>
;¤
*guise.DefaultGuiseApplication:
destinations=
[
*guise.ComponentDestination("helloworld", example.HelloWorldPanel)
]
;
.
If this application was deployed on the web platform on localhost
using the web.xml
file described above, this application description document would allow the user to browse to http://localhost/webapp/example/helloWorld
and be presented with an instance of com.example.HelloWorldPanel
.
Guise Session
A new instance of GuiseSession
will be created for each user that accesses your application. The Guise session keeps track of the state of user interaction, including the locale, the current navigation path, and which principal if any is logged in. The session allows access to resources based upon the current locale, and provides access to the platform if it is ever necessary to know about platform-specific information such as environment variables.